Java中的HashMap是一种基于哈希表的数据结构,用于存储键值对。它实现了Map接口,允许我们通过键来快速查找对应的值,具有高效的插入、删除和查找操作。HashMap内部使用数组和链表(或红黑树)组合的方式来实现,它的核心思想是通过哈希算法将键映射到数组索引上,从而实现快速的查找。
HashMap的原理:
存储结构:HashMap内部维护一个Entry数组,每个Entry包含键、值和指向下一个Entry的指针(链表或红黑树节点)。
哈希计算:当我们插入一个键值对时,首先会对键进行哈希计算,得到一个哈希码。HashMap使用哈希码和数组长度取模的方式来确定该Entry在数组中的位置。
处理哈希冲突:由于不同的键可能映射到相同的数组索引上,这就是哈希冲突。HashMap内部使用链表或红黑树来解决哈希冲突问题,当链表长度超过一定阈值时,链表会转换为红黑树,提高查找效率。
扩容:当HashMap中的元素数量达到负载因子(load factor)与容量的乘积时,HashMap会自动扩容,重新计算每个元素的位置,以保证哈希表的性能。
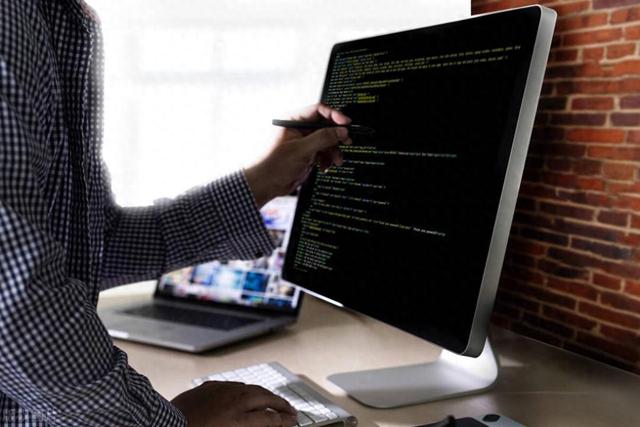
HashMap的使用场景:
高效查找:HashMap适用于需要快速查找特定键对应值的场景,时间复杂度为O(1)。
键值存储:HashMap适合存储键值对数据,比如缓存数据、配置信息等。
数据唯一性:HashMap中的键是唯一的,可以用于去重或判断某个键是否存在。
接下来,我将演示一个简单的自定义HashMap的实际案例。在这个案例中,我将展示如何自己实现一个简单的HashMap,并模拟put和get方法来存储和获取键值对。
import java.util.LinkedList;public CustomHashMap<K, V> { private static final int DEFAULT_CAPACITY = 16; private LinkedList<Entry<K, V>>[] buckets; public CustomHashMap() { buckets = new LinkedList[DEFAULT_CAPACITY]; for (int i = 0; i < DEFAULT_CAPACITY; i++) { buckets[i] = new LinkedList<>(); } } private int getBucketIndex(K key) { return key.hashCode() % DEFAULT_CAPACITY; } public void put(K key, V value) { int index = getBucketIndex(key); LinkedList<Entry<K, V>> bucket = buckets[index]; for (Entry<K, V> entry : bucket) { if (entry.getKey().equals(key)) { entry.setValue(value); return; } } bucket.add(new Entry<>(key, value)); } public V get(K key) { int index = getBucketIndex(key); LinkedList<Entry<K, V>> bucket = buckets[index]; for (Entry<K, V> entry : bucket) { if (entry.getKey().equals(key)) { return entry.getValue(); } } return null; } private static Entry<K, V> { private K key; private V value; public Entry(K key, V value) { this.key = key; this.value = value; } public K getKey() { return key; } public V getValue() { return value; } public void setValue(V value) { this.value = value; } } public static void main(String[] args) { CustomHashMap<String, Integer> map = new CustomHashMap<>(); map.put("one", 1); map.put("two", 2); map.put("three", 3); System.out.println("Value for key 'one': " + map.get("one")); System.out.println("Value for key 'two': " + map.get("two")); System.out.println("Value for key 'three': " + map.get("three")); // Output: // Value for key 'one': 1 // Value for key 'two': 2 // Value for key 'three': 3 }}在上述代码中,我们实现了一个简单的CustomHashMap类,其中包含put和get方法用于存储和获取键值对。我们通过哈希算法确定键值对在数组中的位置,并使用链表来处理哈希冲突。通过这个案例,我们可以更好地理解HashMap的原理和使用方法,并自己动手实现一个简单的HashMap数据结构。